Deep Dive: System Architecture Differences - Linux vs. Windows
Category: Linux
Date: 3 months ago
Views: 528
The Inner Workings of Linux System Calls
For the technically inclined, delving into the core of Linux reveals a design philosophy centered around openness and transparency. At the heart of this lies the system call interface, a set of open and documented entry points that enable user applications to communicate directly with the kernel. In the following example, the C program uses the "write" system call to print "Hello, Linux!" to the standard output:
// Linux System Call Example
#include <stdio.h>
#include <unistd.h>
int main() {
write(1, "Hello, Linux!\n", 13);
return 0;
}
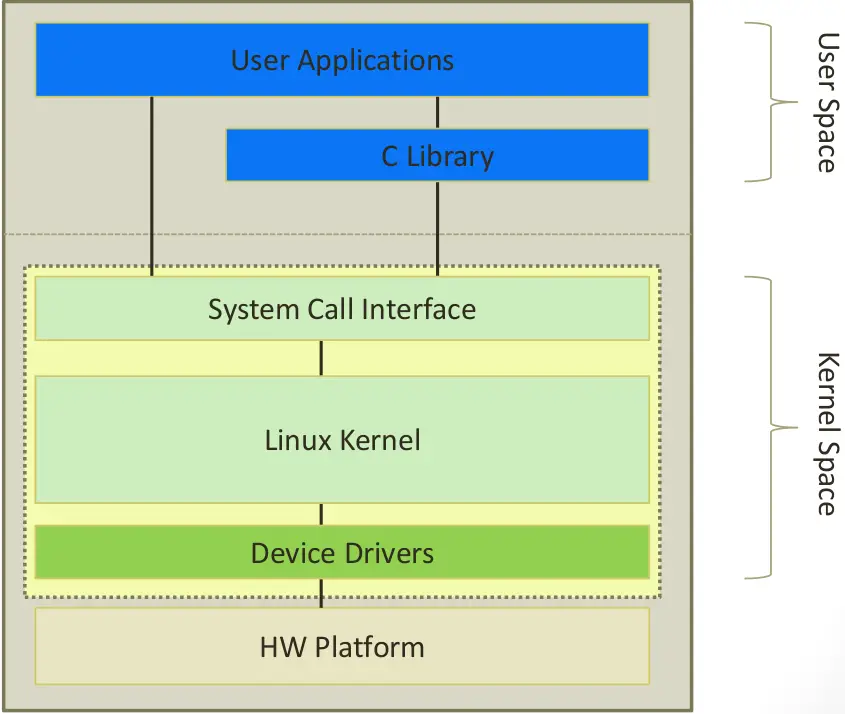
Windows: Navigating Through Layers
Windows, on the other hand, employs a more intricate and layered architecture. As user applications interact with the operating system, they traverse through several intermediary layers. At the forefront is the Windows API (WinAPI), a comprehensive set of functions and procedures. However, beneath this facade, developers encounter layers such as winntdll, which acts as a bridge between user space and the kernel. Consider the following example showcasing the use of WinAPI in a Windows C program:
// Windows WinAPI Example
#include <windows.h>
#include <stdio.h>
int main() {
MessageBox(NULL, "Hello, Windows!", "Message", MB_OK);
return 0;
}
Here, the C program utilizes the "MessageBox
" function provided by the WinAPI. However, this function is just the tip of the iceberg. Under the hood, it calls a series of other functions and passes through layers such as winntdll.
For instance, "MessageBox
" may internally call functions like "CreateWindow
," which in turn involves more intricate processes. The detailed execution path is encapsulated within the closed layers of the Windows architecture.
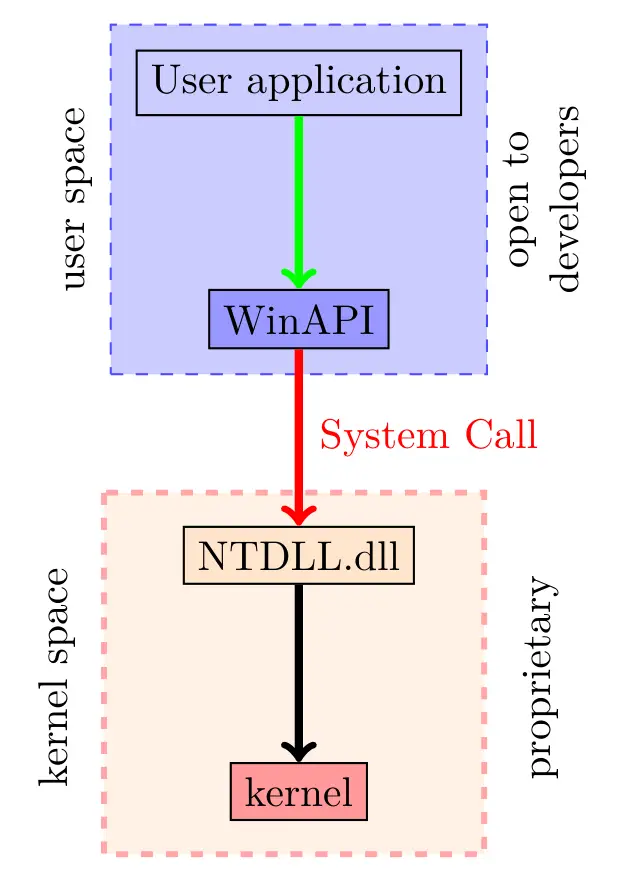
Linux's System Call Interface: A Programmer's Perspective
From a programming standpoint, the direct communication facilitated by Linux's system call interface offers a programmer unparalleled control and visibility. The absence of excessive intermediary layers streamlines the development process, making it easier to understand and optimize system interactions.
Peering Into the Kernel: A Windows Perspective
In contrast, navigating through Windows' layered architecture requires a keen understanding of the interactions between user space and the Windows Executive. The transition from user space to kernel mode involves traversing through proprietary layers, adding intricacy to the debugging and optimization processes.
Conclusion: Unraveling the Architectural Nuances
In conclusion, the architectural disparities between Linux and Windows reveal themselves in the transparency of Linux's system calls and the layered complexity of Windows. For developers and system architects, choosing between the two involves weighing the advantages of a straightforward, open system against the added complexities of a layered architecture.
0 Comments, latest
No comments.